In this quick tutorial, I’ll show you six different approaches to getting the current year in Python. Python is a method and module-rich programming language that provides multiple ways to retrieve the current year.
Category: Python Tutorials
This page will have all tutorials which are related to python 3.
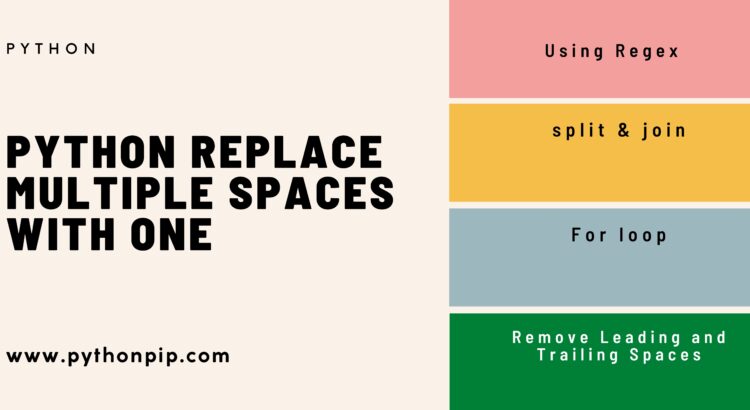
Python Replace Multiple Spaces with One
This quick Python tutorial helps to solve the problem “python replace multiple spaces with one”. We’ll discuss different approaches to solve this issue. There are several scenarios where you have strings that have multiple Whitespace and you want to replace them with a single space using Python.
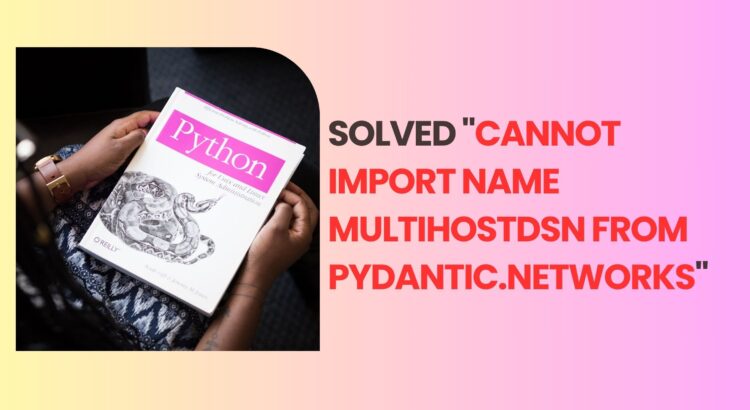
Solved “Cannot Import Name Multihostdsn from pydantic.networks”
In this post, we’ll explore various reasons behind this import error. I’ll walk you through the causes of this error message and how to fix it. This occurs when there is a version mismatch between Pydantic and one of its dependencies.
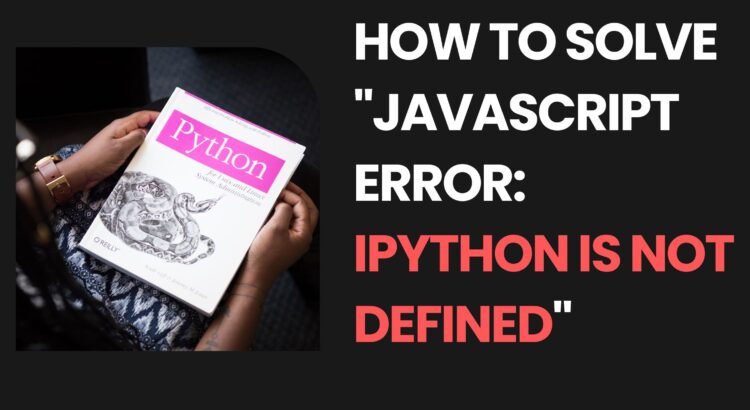
How To Solve “Javascript Error: IPython Is Not Defined”
in this article, We’ll explore the various reasons behind generating “Javascript Error: IPython Is Not Defined” error. I’ll walk you through the causes of this error message and how to fix it.
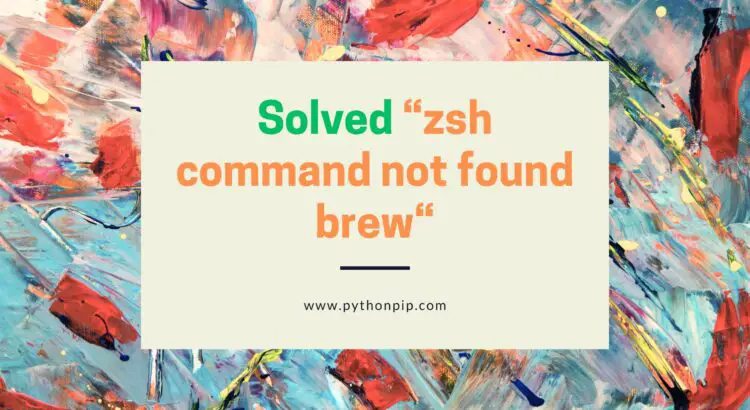
How To Solve “zsh: command not found: brew” in macOS
in this tutorial, We will troubleshoot “zsh: command not found: brew” in the system. This error usually indicates that the Homebrew binary is not in the system’s PATH or that Homebrew is not installed correctly.
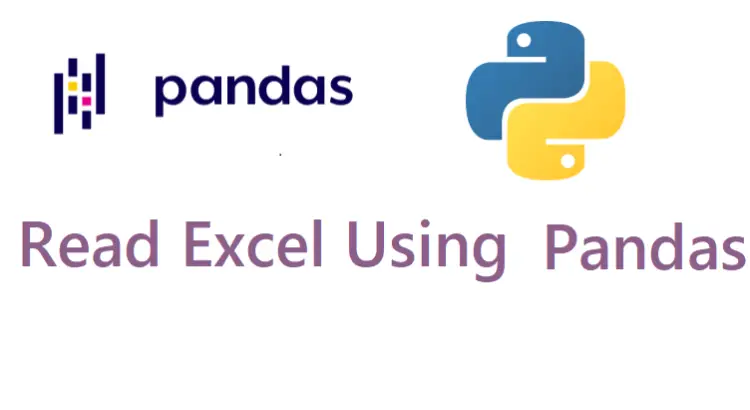
Reading Excel Using Python Pandas
This python tutorial help to read excel file using pandas. The pandas module help to read excel file data using read_excel() function into a DataFrame object. I have already shared tutorial How To Read & Update Excel File Using openpyxl.You will learn here how to read an excel file and display data using pandas. You […]
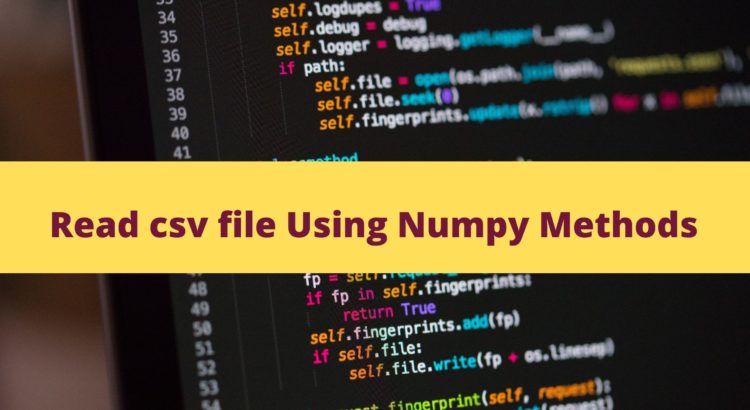
Read CSV file Using Numpy
I’ll show you how to read a csv file and convert it into a NumPy array in this post. We’ll write NumPy data to a CSV file. The CSV file will then be read and transformed to a Numpy array. I’ll show you how to read a CSV file using both numpy.loadtxt() and numpy.genfromtxt() methods. […]
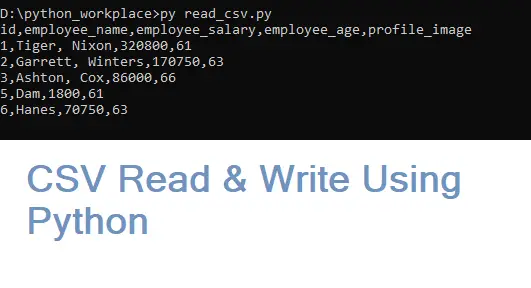
Read and Write CSV Data Using Python
This tutorial will show you how to make a CSV parser in Python 3. The most common file format for storing plain text data is CSV (comma-separated values). It is one of the most widely used data exchange formats between servers. Each data value is separated by a comma in the CSV files. Exchanging information […]
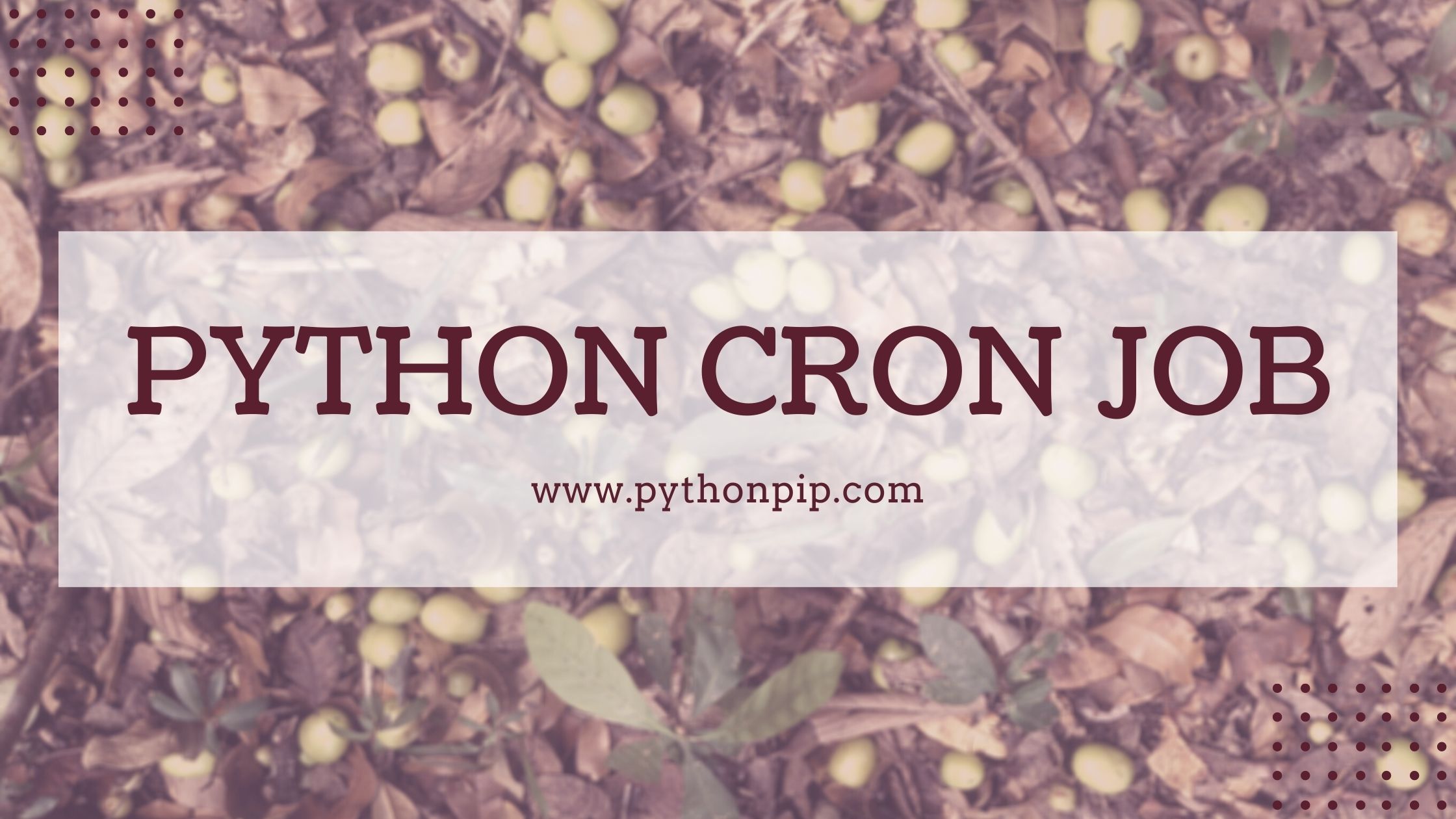
Python Cron job With Example
This tutorial will help to understand python cron jobs with examples. The cron helps to execute a periodic task on the server like sending emails, database cleanup, and generating reports. To automate these tasks we can use Python Cron Job scheduling. We ll learn how to implement cron job scheduler with python. What’s Cron Job […]
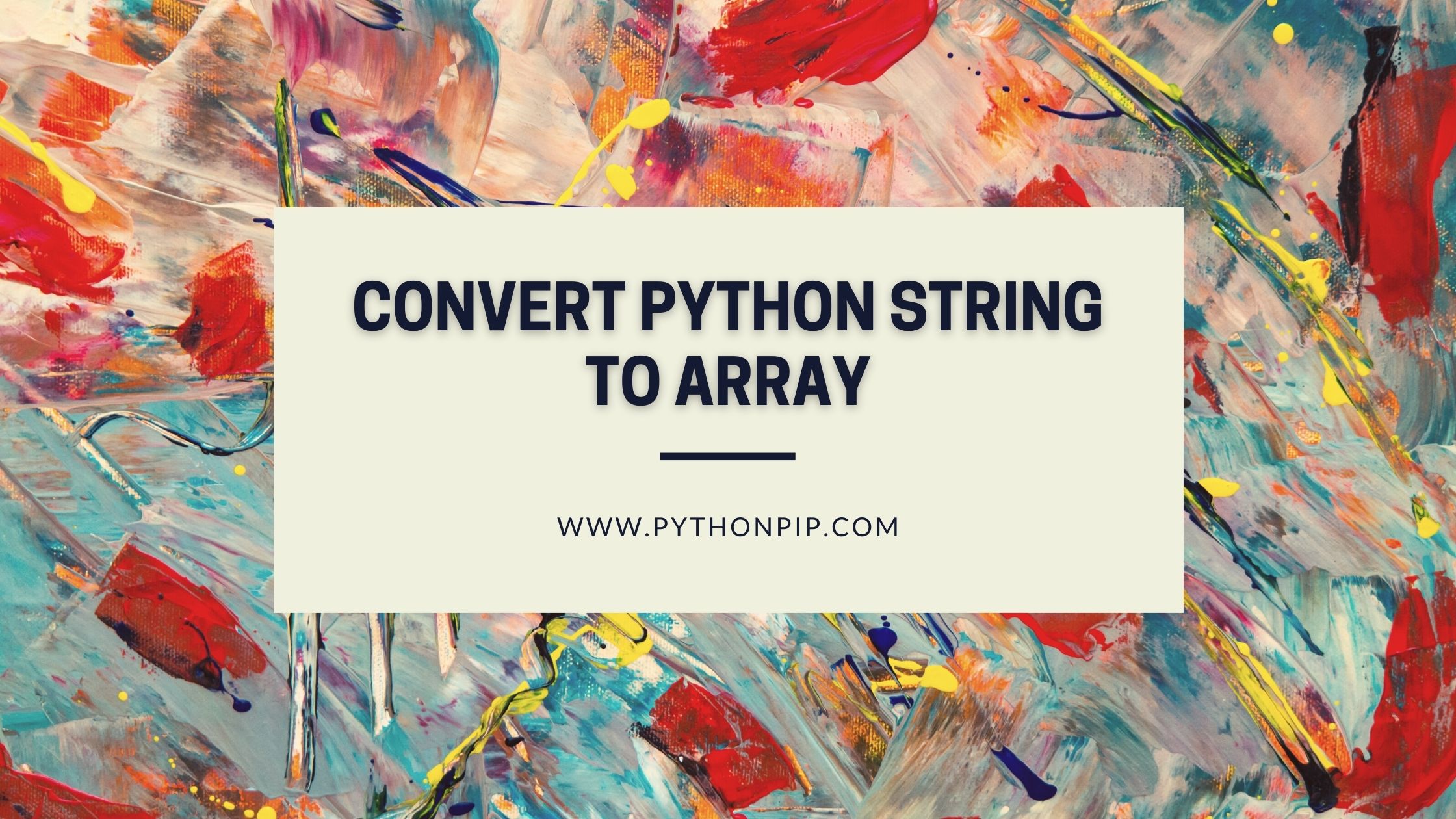
How To Convert Python String To Array
This tutorial help to create python string to array. Python does not have an inbuilt array data type, it has a list data type that can be used as an array type. So basically, we are converting a string into a python list. Python String to Array We’ll convert String to array in Python. The […]