In this article, We will explore how to work with MySQL databases using Python. Also, We’ll learn about the integration of MySQL with Python, allowing you to interact with databases and perform various operations.
Category: Python Tutorials
This page will have all tutorials which are related to python 3.
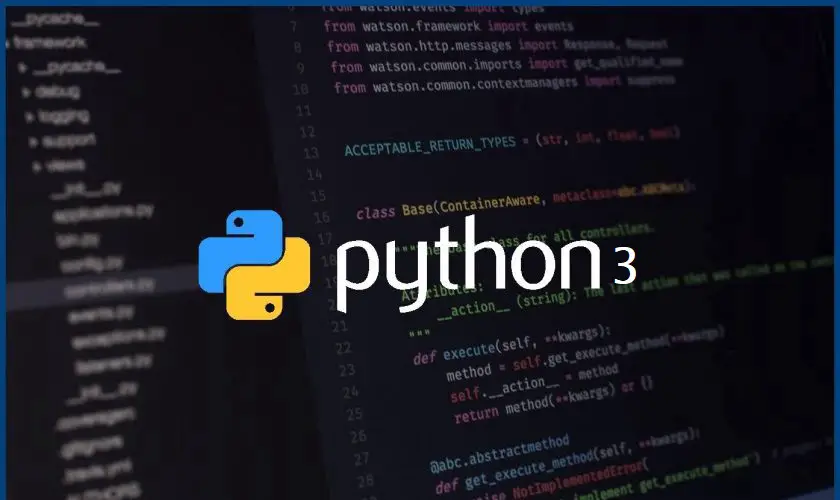
Change default python 2.7 to python 3 in Ubuntu
This is a very common issue for Python beginners, This tutorial helps to change the Python version from python 2.7 to python 3. By default Ubuntu have inbuilt Python 2, We need to upgrade Python 3, there is a difference between python 2 and python 3.
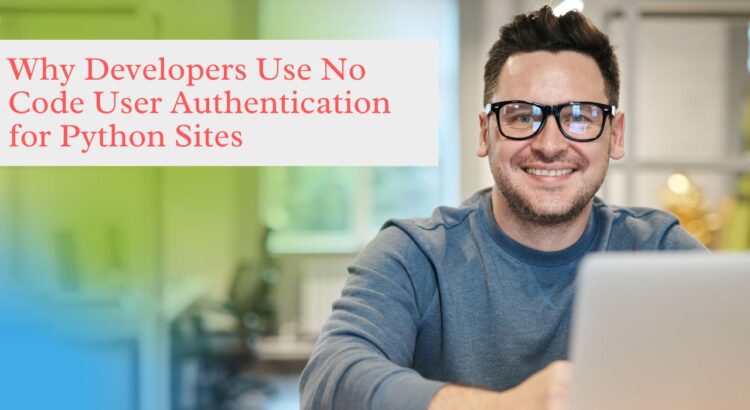
Why Developers Use No Code User Authentication for Python Sites
A lot of businesses these days use Python websites, and many developers use this programming language for the development of websites. It offers a host of benefits and has a solid history and reputation, having been developed in the early 1990s.

XOR in Python with Example
The XOR operator, also known as the exclusive or operator, is a bitwise operator used in Python to perform logical operations on binary values. This article explains how to use the XOR operator in Python with code examples. We will look to explore multiple ways to perform XOR (exclusive OR) operations in Python with examples. […]
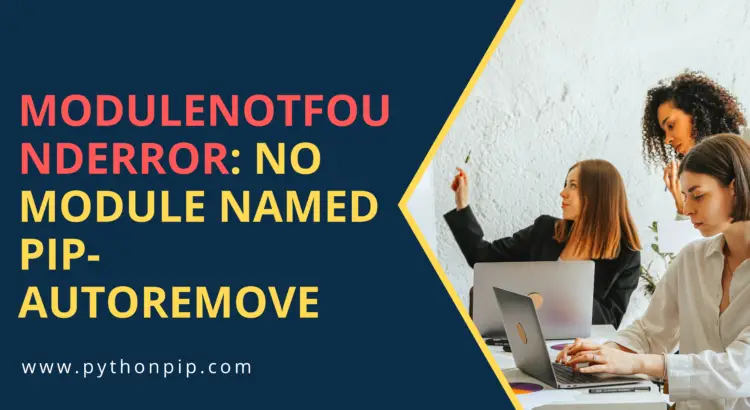
ModuleNotFoundError: No module named pip-autoremove
The pip-autoremove is a Python package that enables you to remove unnecessary packages that have been installed as dependencies for other packages. In this article, we’ll explore what causes this error and how you can fix it.
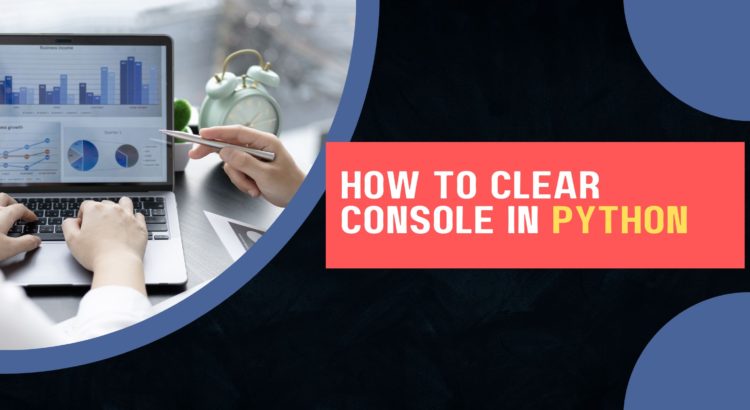
How to Clear Console in Python
This tutorial helps How to Clear the Console in Python. There is a number of ways to clear the console based on the operating system. You can also clear the interpreter programmatically. There are several methods for clearing the console in Python, depending on the operating system you are using.
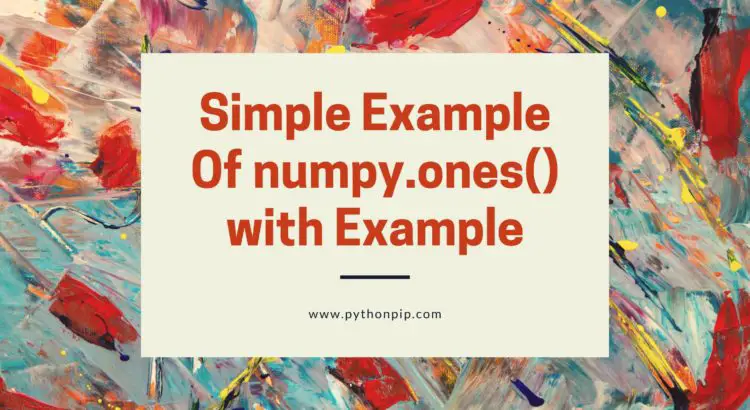
What is numpy.ones() and uses
The np.ones() function returns a one-dimensional matrix. It can be used to initialize the weights in TensorFlow and other statistical tasks during the first iteration.
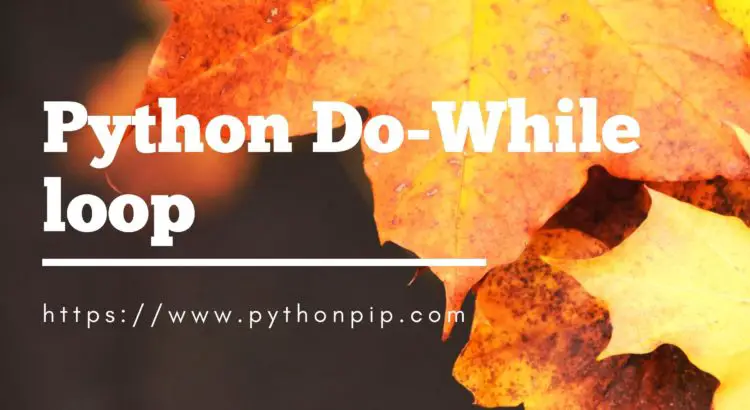
Python do while with Example
Python doesn’t have do-while loop. However, you can achieve a similar effect by using a while loop and a break statement. This Example helps to create a program that helps to execute ‘do while loop’ with an example. There are no of loops available to iterate over elements/items but do-while does not have.
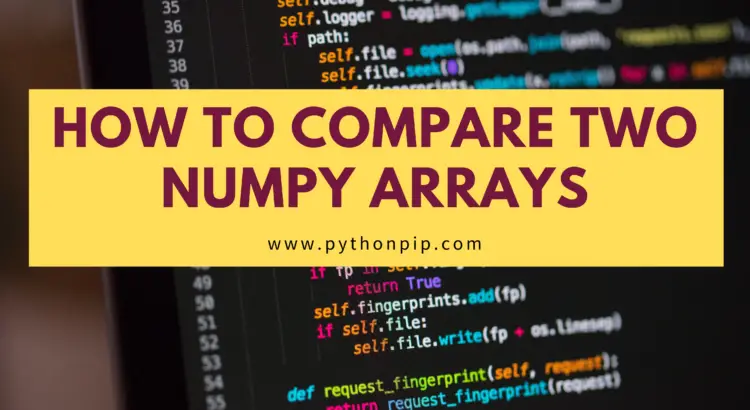
How To Compare Two Numpy Arrays
This python article focuses on comparisons between two arrays performed with NumPy. When two NumPy arrays are compared, every element at each corresponding index is checked to see if they are equivalent.
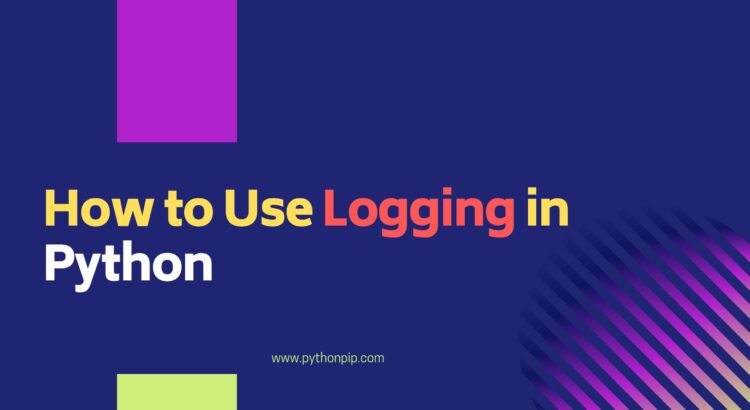
How to Use Logging in Python
A built-in module called Python log defines the classes and functions that implement the adaptable event-logging system for libraries and applications. Logging is the process of storing information in a log file or printing into stdout, which can then be utilized for debugging if a problem arises.