This python tutorial helps to understand Python’s zip() function with examples. The zip() is a built-in Python function that accepts any type of iterable and returns us an iterator of tuples. This method creates an iterator that will aggregate elements from two or more iterables. This built-in function creates an iterator that aggregates elements from […]
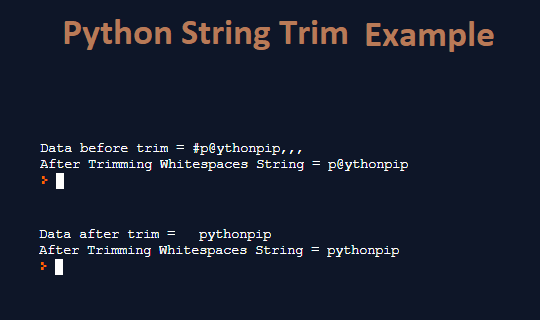
How to Trim Python string
This Python 3 tutorial will show you how to trim strings. I’ll go over some scenarios for trimming strings in Python. Trimming the string helps to remove the white gaps. The leading and following spaces in a Python string can be removed. The python has string package to handle string manipulation. You can also checkout […]
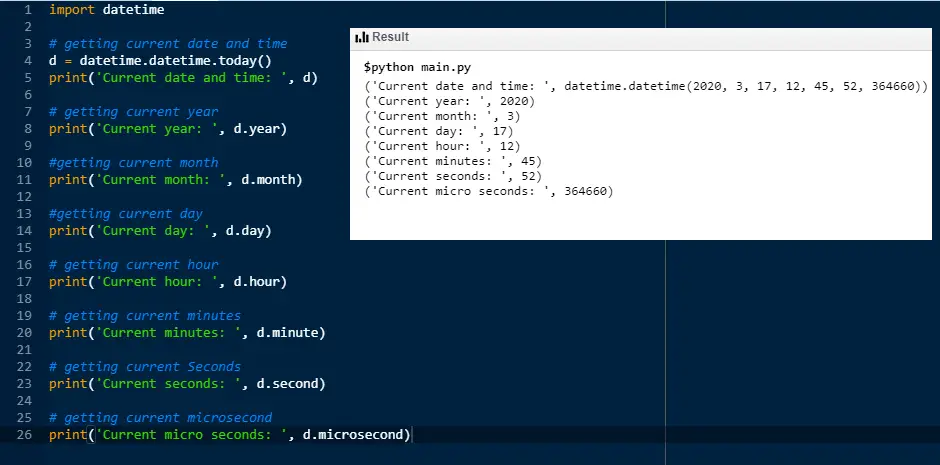
Get Current Date and Time with Examples
This python tutorial help to get the current date using python 3. The Python datetime class can be used to get and manipulate date and time. We will also format the date and time in different formats using strftime() method.
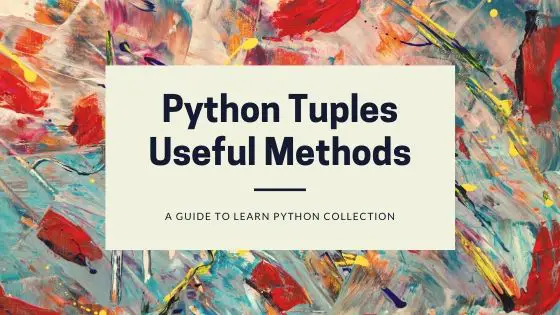
Python Tuple Example & Methods
This tutorial helps to understand Python tuple with examples. It’s a very common and useful collection types. A tuple is a collection that is ordered and unchangeable. How To Define Tuple in Python Python tuples are written with round brackets. Access Tuple Items We can access tuple items by referring to the index number of […]
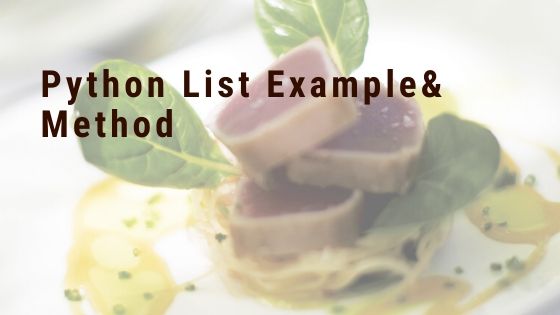
Python List Example And Methods
This python tutorial help to understand the list and useful methods. The list is the most popular and versatile datatype used in Python. It’s the same as an array as like other programming languages.
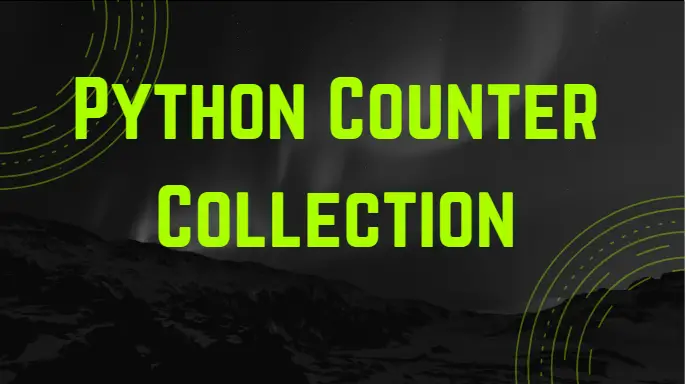
Python Collections Counter Example
Python Counter class is part of Collections module. Counter is used to keep track of elements and their count. The Counter is an unordered collection where elements are stored as dict keys and their count as dict value. You can store positive, zero or negative integers into the counter. We can also store objects too […]
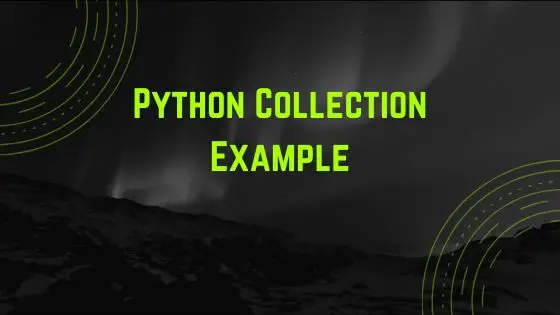
Python Collection Example
This tutorial help to understand python collection types. There are four collection data types in python. It’s a very common and useful data type for python programming language.
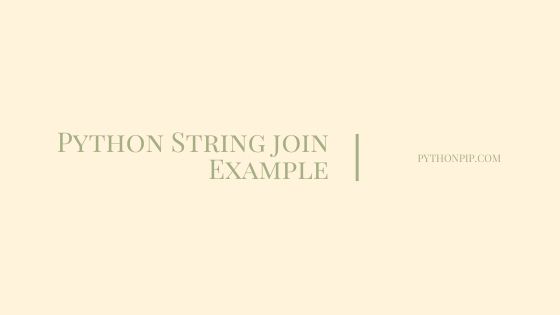
Python String join Example
This Python tutorial will show you how to join strings arrays. The string method join() returns a string concatenated with the elements of an iterable. It concatenates each element of an iterable (such as a list, string, or tuple) to the string and returns the result. The syntax of join() is: If the iterable contains […]
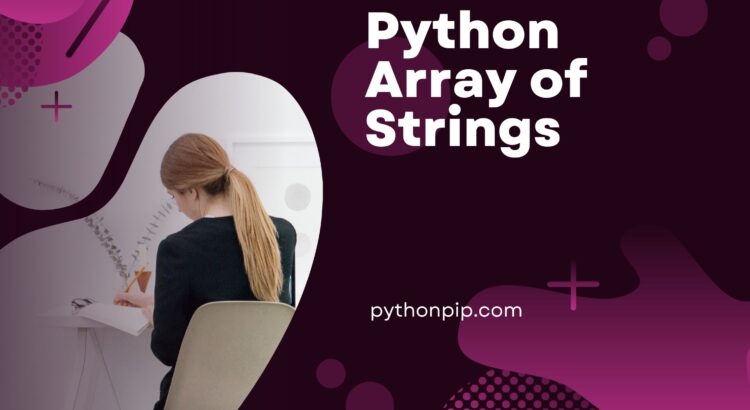
Python Array of Strings
This tutorial helps to create python array of strings. Python does not have built-in support for Arrays. Python lists are used to create an array using capacity. An array is a collection of elements of the same type.
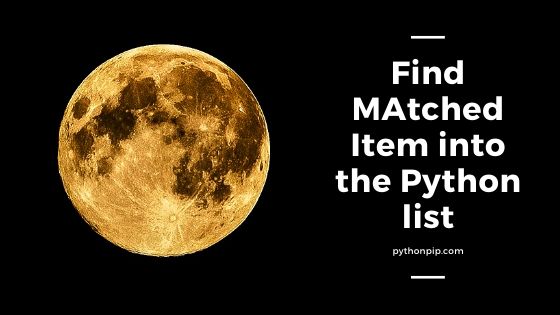
How To Match String Item into List Python
This tutorial help to find python list item contains a string. I’ll walk you through the many circumstances for finding items in a Python list.. I have also shared How To Find Substring and Character into String. I’ve given an example utilizing the find() method, as well as the operator and index functions. You can […]